Cool new features in Python 3.6
Python 3.6 adds a couple of new features and improvements that’ll affect the day to day work of Python coders. In this article I’ll give you an overview of the new features I found the most interesting.
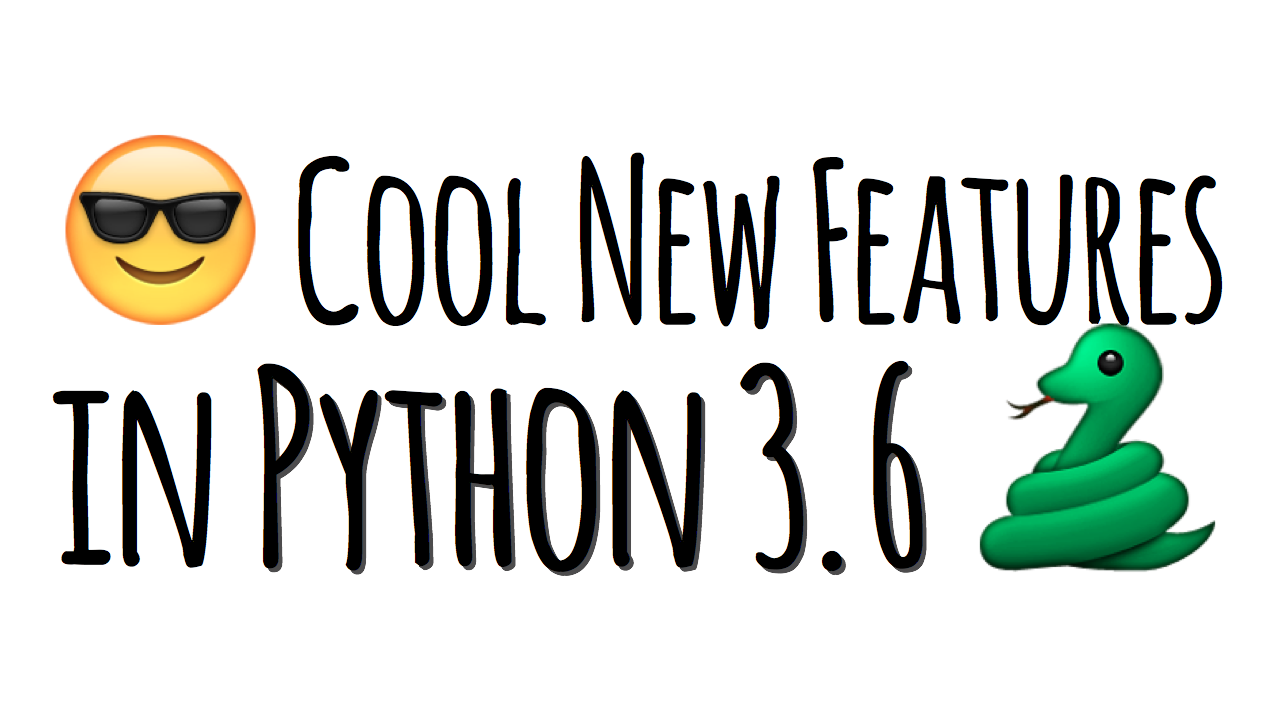
Improved numeric literals
This is a syntactic tweak that makes numeric literals easier to read. You can now add underscores to numbers in order to group them to your liking. This is handy for expressing large quantities or constants in binary or hexadecimal:
>>> six_figures = 100_000 >>> six_figures 100000
>>> programmer_error = 0xbad_c0ffee >>> flags = 0b_0111_0101_0001_0101
Remember, this change doesn’t introduce any new semantics. It’s just a way to represent numeric literals differently in your source code. A small but neat addition.
You can learn more about this change in PEP 515.
String interpolation
Python 3.6 adds yet another way to format strings called Formatted String Literals. This new way of formatting strings lets you use embedded Python expressions inside string constants. Here’s are two simple examples to give you a feel for the feature:
>>> name = 'Bob' >>> f'Hello, {name}!' 'Hello, Bob!'
>>> a = 5 >>> b = 10 >>> f'Five plus ten is {a + b} and not {2 * (a + b)}.' 'Five plus ten is 15 and not 30.'
String literals also support the existing format string syntax of the str.format()
method. That allows you to do things like:
>>> error = 50159747054 >>> f'Programmer Error: {error:#x}' 'Programmer Error: 0xbadc0ffee'
Python’s new Formatted String Literals are similar to the JavaScript Template Literals added in ES2015/ES6. I think they’re quite a nice addition to the language and I look forward to using them in my day to day work.
You can learn more about this change in PEP 498.
Type annotations for variables
Starting with Python 3.5 you could add type annotations to functions and methods:
>>> def my_add(a: int, b: int) -> int: ... return a + b
In Python 3.6 you can use a syntax similar to type annotations for function arguments to type-hint standalone variables:
>>> python_version : float = 3.6
Nothing has changed in terms of the semantics–CPython simply records the type as a type annotation but doesn’t validate or check types in any way.
Type-checking is purely optional and you’ll need a tool like Mypy for that, which basically works like a code linter.
You can learn more about this change in PEP 526.
Watch a video summary of the best new features in Python 3.6
» Subscribe to the dbader.org YouTube Channel for more Python tutorials.
Other notable changes
I think Python 3.6 will be an interesting release. There are many more interesting additions and improvements that are worth checking out. You can learn more about them in the links below or by reading the official “What’s New In Python 3.6” announcement.