Fundamental Data Structures in Python
In this article series we’ll take a tour of some fundamental data structures and implementations of abstract data types (ADTs) available in Python’s standard library.
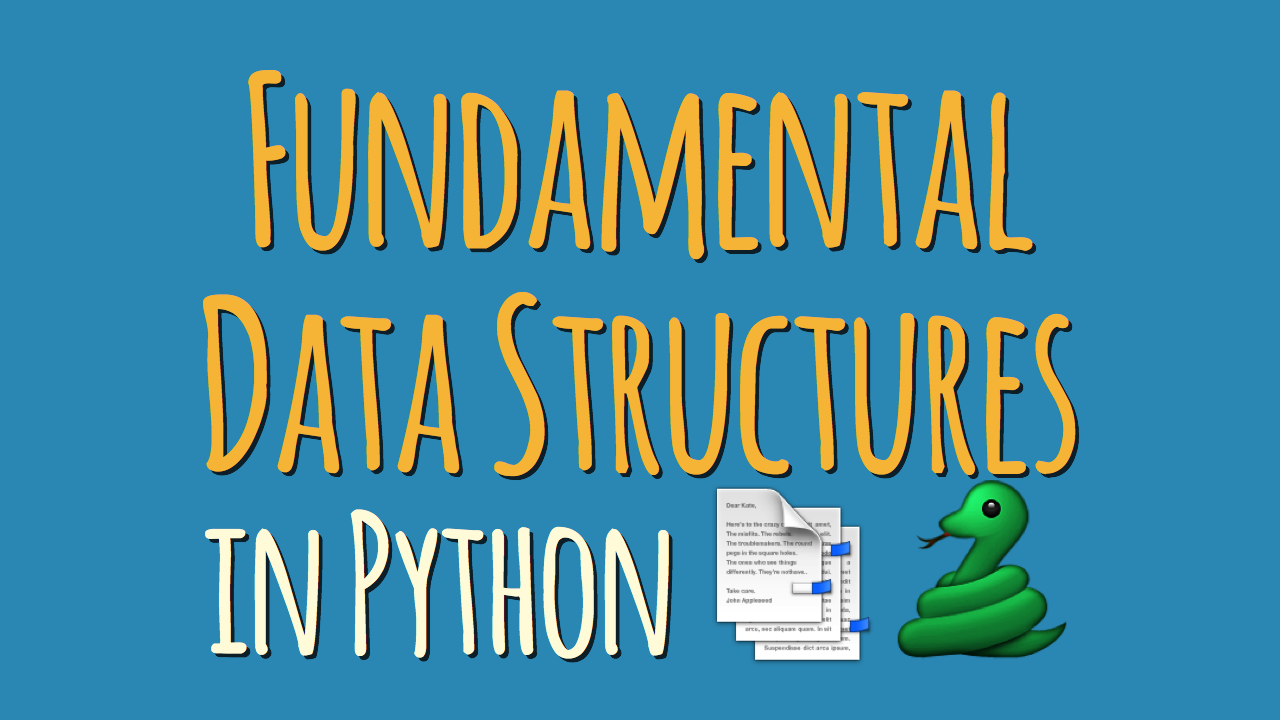
Data structures are the fundamental constructs around which you build your applications. Each data structure provides a particular way of organizing data so it can be accessed efficiently, depending on the use case at hand.
Python ships with an extensive set of data structures in its standard library. However due to naming differences it’s often unclear how even well-known “abstract data types” correspond to a specific implementation in Python.
Other languages like Java stick to a more “computer sciencey” and explicit naming scheme for their standard data structures. For example, a list isn’t just a “list” in Java—it’s either a LinkedList
or an ArrayList
. This makes it easier to recognize the computational complexity of these types.
Python favors a simpler and more “human” naming scheme. The downside is that to a Python initiate it’s unclear whether the built-in list
type is implemented as a linked list or a dynamic array.
My goal with this article series is to clarify how the most common abstract data types map to Python’s naming scheme and to provide a brief description for each. This information will also help you in Python coding interviews.
If you’re looking for a good book to brush up your data structures knowledge I highly recommend Steven S. Skiena’s The Algorithm Design Manual.
It strikes a great balance between teaching you fundamental (and more advanced) data structures and then showing you how to put them to practical use in various algorithms. Steve’s book was a great help in the writing of this series.
Alright, let’s get started. This article serves as the “hub” for the individual data structure tutorials that I’ll link in the list below:
Python Data Structures Tutorials
- Dictionaries, Maps, and Hash Tables in Python
- Sets and Multisets in Python
- Arrays in Python
- Records, Structs, and Data Transfer Objects in Python
- Stacks in Python
- Queues in Python
- Priority Queues in Python
- Linked Lists in Python
By the way, I’m always looking to improve these tutorials so if you find an error or would like to suggest an addition—please leave a comment on the article or reach out to me via email or Twitter.