Let’s Program with Python: Reacting to User Input (Part 4)
In the fourth (and final) class in this series you’ll learn how to make your Python programs interactive by letting them react to user input.
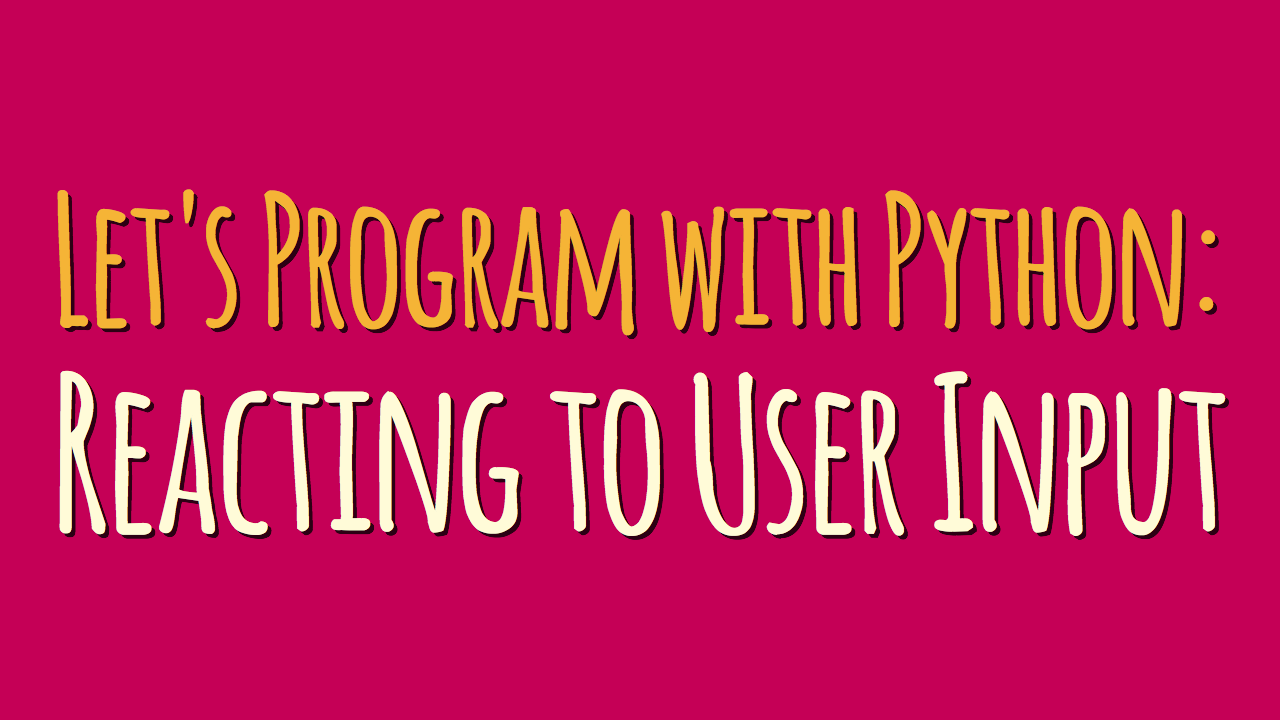
In this guest post series by Doug Farrell you’ll learn the basics of programming with Python from scratch. If you’ve never programmed before or need a fun little class to work through with your kids, you’re welcome to follow along.
Looking for the rest of the “Let’s Program with Python” series? Here you go:
- Part 1: Statements, Variables, and Loops
- Part 2: Functions and Lists
- Part 3: Conditionals and “if” Statements
- Part 4: Reacting to User Input (This article)
Table of Contents – Part 4
- Let’s Write a Program Together
- Getting Information From the Player
- Converting a String to a Number
- Another Kind of Loop
- More Things We Can Do With Lists
- How Many Items Are in a List?
- How to Pick Random Things From a List?
- Our Completed “Guess My Number” Program
- Congratulations!
- Appendix – Python Info That Doesn’t Fit in Class
Let’s Write a Program Together
For this class we’re going to write a “Guess My Number” game program. In this game the program will pick a random number from 1 to 10 and the player will try to guess what the number is. The program will respond in different ways depending on whether the player guessed correctly or incorrectly. The player can also end the game whenever they want by telling the program to “quit”.
The interesting part of this program is you’re going to tell me how to write it instead of the other way around. But before we get started, we need to learn a few more things about Python to help us build our game.
Getting Information From the Player
In order to play our game the player has to interact with it. We need a way to get guesses from the player so the game can compare its secret number to the players guess. To do this we use the input()
function.
The input()
function let’s us ask the user for some information, and then wait for them to enter something using the keyboard. In the Python interactive mode it looks like this:
>>> guess = input("Please enter a number: ") Please enter a number:
At the point where the input()
function runs, the cursor is at the end of the "Please enter a number: "
string, waiting for you to type something.
You can type anything you want, when you hit the <ENTER>
key whatever you typed will be assigned to the guess
variable as a string. This is a very simple way to get input from the user using the keyboard.
Converting a String to a Number
We haven’t talked about this yet, but there is a difference between a string like "10"
and the number 10
. Try this in the interactive mode:
>>> 10 == 10 True >>> "10" == 10 False
On the first line we are comparing the two number 10’s to each other to see if they are equal. Python knows they are, so it responds by printing True
to the screen.
But the next comparison, "10" == 10
, why does Python respond with False
? The simple answer is Python doesn’t think they’re equal.
But why aren’t they equal? This can be confusing, "10"
looks like the number ten. And 10
definitely looks like the number ten as well. For Python however, this isn’t true.
The number 10
is exactly that, the numerical value 10. The string "10"
is just a string, it has no numerical value, even though "10"
looks like ten to us.
The difference is the representation. The "10"
represents a string to Python, it doesn’t know that string represents ten to us. The 10
however does mean numerical ten to Python, ten things, ten cars, ten whatever.
What does this have to do with our game? A lot actually. When the game starts the program will randomly pick a number from 1 to 10, not a string, a number. However when the player types something into our guess = input("Please enter a number: ")
prompt, guess
is a string variable.
Even if the player enters a “1” and then a “0” and then hits enter, the guess
variable will be a string. This is where a problem comes in. Let’s say we call the game’s variable for its number secret_number
. If we write some Python code that compares them, like this:
if secret_number == guess:
This code will fail because comparing a string to a number will always be False
. We need to make Python compare two of the same kinds of things. For our game, both things need to be numbers. We need to convert the player’s guess
variable to a number. Python can do this using the int()
function. It looks like this:
guess_number = int(guess)
With this code we’re taking the player’s input, guess
, which could be something like “8”, and converting it to the numerical value 8 and assigning it to the new variable guess_number
. Now when we compare guess_number
with secret_number
, they are the same kind of thing (numbers) and will compare correctly when we write Python code like this:
if guess_number == secret_number:
Another Kind of Loop
We’ve only used the for
loop so far because it’s handy when you know ahead of time how many times you want to loop. For our game program we won’t know ahead of time how many guesses it will take our player to guess the secret_number
. We also don’t know how many times they’ll want to play the game.
This is a perfect use for the other loop Python supports, the while
loop. The while
loop is called a conditional loop because it will continue looping until some condition it is testing is True. Here’s an example of a while
loop:
game_running = True while game_running: # Run some Python statements
What these program lines mean is that while the variable game_running
is True
, the while loop will keep looping. This also means something in the while
loop will have to change the value of game_running
in order for the program to exit the loop.
Forgetting to provide a way for the while
loop to end creates what’s called an infinite loop. This is usually a bad thing and means in order to exit the program it has to be crashed or stopped in some other way.
More Things We Can Do With Lists
We’ve used Python lists before to hold things we want to deal with as one thing, like lists of turtles. We’ve created lists and appended things to lists. So far we’ve used the things in the list one at a time using the for
loop. But how do we get to the individual things inside a list? For example, suppose I have this list in Python:
names = ["Andy", "George", "Sally", "Sharon", "Sam", "Chris"]
How can I get just the "Sally"
name from the names
list variable? We use something called list indexing to do that. Everything in a list has a position in the list, and all lists in Python start at position 0. The position is called an index, so to get "Sally"
from the list, remembering all lists start at index 0, we do this:
name = names[2]
When we do this the variable name
will be equal to "Sally"
from our list. The [2]
above is called the index into the list. We’ve told Python we want the thing inside the names
list at index 2.
How Many Items Are in a List?
It’s often useful to be able to find out how many things are in a list. For instance, our names
list above has six strings in it. But how could we find this out using Python? We use the len()
function. It looks like this:
number_of_names_in_list = len(names)
This will set the variable number_of_names_in_list
equal to six. Notice something about the number of items in the names
list and the largest index, the name “Chris”. To get the name “Chris” from our names
list we would do this:
name = names[5]
The last thing in the list is at index 5, but the number of things in the list is 6. This is because all lists start with index 0, which is included in the number of things in the list. So for the names list we have indexes 0, 1, 2, 3, 4 and 5, totaling 6 things.
How to Pick Random Things From a List?
Now we know how to pick individual things from a list, how to determine how long a list is and what the maximum index value in a list is. Can we use this information to choose a random thing from a list? For a minute let’s think about our turtle programs, we had a list something like this:
colors = ["black", "red", "organge", "yellow", "green", "blue"]
How could we pick a random color from this list to use when we were creating a turtle? We know the smallest index is 0, which would be the color “black”. We also know by looking at the list that our largest index is 5, the color blue. This is one less than the number of colors in the list. So we could do something like this:
colors = ["black", "red", "organge", "yellow", "green", "blue"] turtle_color = colors[random.randint(0, 5)]
This Python statement would set the turtle_color
variable to a random color from our colors
list. But what if we added more colors to our list? Something like this:
colors = ["black", "red", "organge", "yellow", "green", "blue", "violet", "pink"] turtle_color = colors[random.randint(0, 5)]
Unless we change the 5
in the random.randint(5)
function we’ll still be picking from the first six colors and ignoring the new ones we added. What if we’re picking random colors all over our program, we’d have to change all the lines that pick a color every time we changed the number of colors in our colors
list. Can we get Python to handle this for us? Sure we can, we can use the len()
function to help us out. We can change our code to look like this:
colors = ["black", "red", "organge", "yellow", "green", "blue", "violet", "pink"] turtle_color = colors[random.randint(0, len(colors) - 1)]
What’s going on here? We still have our colors
list variable, but now we’re using the len()
function inside our random.randint()
function. This is okay, the len()
function returns a number and random.randint()
expects a number as its second parameter.
But now we’re telling random.randint()
the upper index limit of the numbers we want to choose from is one less than the number of things in the colors
list variable. And as we’ve seen, one less than the number of things in a list will always be the highest index in the list. By using the code above we can add or subtract as many items from the colors
list as we want and our random selection will still work, using all the things in the list.
Our Completed “Guess My Number” Program
Here’s our Guess My Number program, complete with comments:
# # Guess My Number # import random # Set our game ending flag to False game_running = True while game_running: # Greet the user to our game print() print("I'm thinking of a number between 1 and 10, can you guess it?") # Have the program pick a random number between 1 and 10 secret_number = random.randint(0, 10) # Set the player's guess number to something outside the range guess_number = -1 # Loop until the player guesses our number while guess_number != secret_number: # Get the player's guess from the player print() guess = input("Please enter a number: ") # Does the user want to quit playing? if guess == "quit": game_running = False break # Otherwise, nope, player wants to keep going else: # Convert the players guess from a string to an integer guess_number = int(guess) # Did the player guess the program's number? if guess_number == secret_number: print() print("Congratulations, you guessed my number!") # Otherwise, whoops, nope, go around again else: print() print("Oh, to bad, that's not my number...") # Say goodbye to the player print() print("Thanks for playing!")
Congratulations!
We’ve completed our course and I hope you’ve had as much fun as I had! We’ve written some pretty amazing programs together and learned quite a bit about programming and Python along the way. My wish is this interested you enough to keep learning about programming and to continue on to discover new things you can do with Python.
Appendix – Python Info That Doesn’t Fit in Class
Differences Between Python and Other Languages
There are many programming languages out in the wild you can use to program a computer. Some have been around for a long time, like Fortran and C, and some are quite new, like Dart or Go. Python falls in the middle ground of being fairly new, but quite mature.
Why would a programmer choose one language to learn over another? That’s a somewhat complicated question as most languages will allow you to do anything you want. However it can be difficult to express what you want to do with a particular language instead of something else.
For instance, Fortran excels at computation and in fact it’s name comes from Fromula Translation (ForTran). However it’s not known as a great language if you need to do a lot of string/text manipulation. The C programming language is a great language if your goal is to maximize the performance of your program. If you program it well you can create extremely fast programs. Notice I said “if you program it well”, if you don’t you can completely crash not only your program, but perhaps even your computer. The C language doesn’t hold your hand to prevent you from doing things that could be bad for your program.
In addition to how well a language fits the problem you’re trying to solve, it might not be able to be used with the tools you like, or might not provide the tools you need, a particular language just may not appeal to you visually and appear ugly to you.
My choice of teaching Python fits a “sweet spot” for me. It’s fast enough to create the kinds of programs I want to create. It’s visually very appealing to me, and the grammar and syntax of the language fit the way I want to express the problems I’m trying to solve.
Python Vocabulary
Let’s talk about some of the vocabulary used in the class and what it means. Programming languages have their own “jargon”, or words, meaning specific things to programmers and that language. Here are some terms we’ve used in relation to Python.
IDLE – command prompt: IDLE is the programming environment that comes with Python. It’s what’s called an IDE, or Integrated Development Environment, and pulls together some useful things to help write Python programs. When you start IDLE it opens up a window that has the Python interactive prompt >>>
in it.
This is a window running the Python interpreter in interactive mode. This is where you can play around with some simple Python program statements. It’s kind of a sandbox where you can try things out. However, there is no way to save or edit your work; once the Python interpreter runs your statements, they’re gone.
IDLE – editor window: The file window (File → New Window) opens up a simple text editor. This is like Notepad in Windows, except it knows about Python code, how to format it and colorizes the text. This is where you can write, edit and save your work and run it again later. When you run this code, behind the scenes IDLE is running the program in the Python interpreter, just like it is in the first IDLE window.
Syntax Highlighting: When we edit code in the file window of IDLE it knows about Python code. One of the things this means is the editor can “colorize”, or syntax highlight, various parts of the Python code you’re entering. It sets the keywords of Python, like for and if, to certain colors. Strings to other colors and comments to another. This is just the file window being helpful and providing syntax highlighting to make it easier for the programmer to read and understand what’s going on in the program.
Python Command Line: In Windows if you open up a command line window, what used to be called a DOS box, and run python, the system will respond with the Python command prompt >>>
. At this point you’re running Python in it’s interactive mode, just like when you’re inside of IDLE. In fact they are the same thing, IDLE is running it’s own Python command line inside the window, they are functionally identical.
You might think “what use is that?”, and I agree, I’d rather work in IDLE if I’m going to using the interactive mode and play with the sandbox mode and the >>>
command prompt. The real use of the Python command line is when you enter something like this at the system command prompt:
python myprogram.py
If I’ve written a program called myprogram.py
and entered the line above, instead of going into interactive mode, Python will read myprogram.py
and run the code. This is very useful if you’re written a program you want to use and not run inside of IDLE. As a programmer I run programs in this manner all day long, and in many cases these programs run essentially forever as servers.
Attribute and Property: We’ve thrown around the terms “attribute” and “property” kind of randomly, and this can lead to some confusion. The reason it’s confusing is these things mean essentially the same thing. When talking about programming there is always the goal to use specific words and terms to eliminate confusion about what you’re talking about.
For instance let’s talk about you. You have many qualities that different people want to express. Your friends want to know your name and phone number. Your school wants to know that as well, and your age, the grade you’re in and our attendance record. In programming terms we can think of these as attributes or properties about you.
The attributes and properties of a thing (you for example) help get more specific information about the thing. And the specific information wanted depends on the audience asking. For example when meeting someone new they are more likely to be interested in your name property. Whereas your school might be more interested in your attendance property.
In Python we’ve been working with turtles, and those turtles have attributes and properties. For example a turtle as a property called forward. This property happens to be a function that moves the turtle forward, but it’s still a property of the turtle. In fact all the properties and attributes associated with a turtle are expressed as functions. These functions either make the turtle do something, or tell us something about the turtle.
Attributes and properties lead into a concept of Object Oriented Programming (OOP) that adds the concept of “things” to programs rather than just data and statements. Object Oriented Programming is beyond the scope of this book, but is very interesting and useful.
Interpreter vs Compiler
In class you’ve heard me talk about the Python interpreter, what does this mean. As we’ve talked about, computer languages are a way for people to tell a computer what to do. But the truth is a computer only understands 0’s and 1’s, so how does a computer understand a language like Python? That’s where a translation layer comes into play, and that translation layer is the interpreter for Python (and other interpreted languages) and a compiler for compiled languages. Let’s talk about compilers first.
Compiler: A compiler is a translator that converts a computer language into machine code, the 0’s and 1’s a computer understands. A compiler usually produces an executable file, on Windows machines this is a file that ends in .exe. This file contains machine code information the computer can run directly. Languages like C, C++ and Fortran are compiled languages and have to be processed by a compiler before the program can run. One thing this means is you can’t run a compiled language directly, you have to compile it first. It also means there is nothing like the interactive mode (the >>>
prompt in Python) in a compiled language. The entire program has to be compiled, it can’t compile and run single statements.
Interpreter: Here’s where things get a little more confusing. Most interpreted languages also have a compiled step, but the output of that step isn’t machine code, no 0’s and 1’s. Instead the compilation step produces what is called ByteCode. The ByteCode is kind of an intermediate step between the near English computer language and the machine code understood by the computer.
The ByteCode can’t be run directly, it is run by a thing called a virtual machine. When the program is run, the virtual machine reads the ByteCode and it generates the computer specific machine code that actually is run by the computer. When you run the program the virtual machine is constantly “interpreting” the ByteCode and generating computer specific machine code. Unlike a compiled language, languages like Python with virtual machines can provide an interactive mode (the >>>
prompt) as the interpreter and virtual machine can translate and run program statements on the fly.
Advantages And Disadvantages: So why would a programmer pick a compiled language over an interpreted language, and vice versa? Well, what we said before still applies, expressiveness of the language, style, etc, those are important things to think about when choosing a language for a project. But there are some differences beyond that. In general compiled languages produce programs that run faster than programs produced by an interpreter. Remember, compiled languages produce programs containing machine code that can be run directly, whereas interpreted languages usually have a virtual machine between the ByteCode and the machine code, so there’s a speed penalty there. However, also keep in mind modern computers are so fast this difference is less important. In addition, interpreted languages are being constantly improved so their performance gets better and better, so the performance difference between the two is shrinking.
Most interpreted languages also offer safety features to prevent the programmer from crashing the program. Interpreted languages make it hard to corrupt memory. They make it difficult to get direct access to the hardware. They don’t force the programmer to manage memory explicitly. Compiled programs like C offer none of this, and therefore it’s easy to do all of those things, which can put your program at risk, unless you’re a skilled programmer. The safety features can be added to a C program, but this has to be done manually by the programmer and isn’t handled by the language natively.
Python Reference Materials
Included below is a list of reference materials to help you go further in your study of Python.
- Python Website – Main Python website
- Python Documentation – Official Python 3 Documentation
- Python Turtle Documentation – Official Python Documentation for Turtle
- Python Tutorials for Beginners on dbader.org
- Learn Python – An interesting tutorial to help learn Python
- How To Think Like A Computer Scientist – Interesting and interactive way to learn Python
- PyGame – An add on module for writing games with Python